简单工厂模式
1.定义
简单工厂模式是属于创建型模式,又叫做静态工厂方法(Static Factory Method)模式,简而言之就是提供一个工厂接收不同的参数来提供不同的对象。
2.具体实现
2.1类图
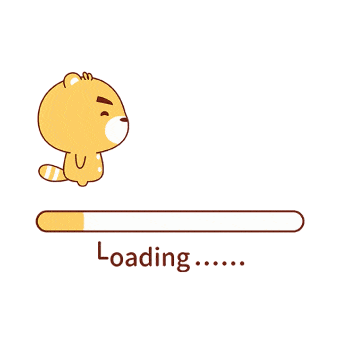
2.2 代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| package 设计模式;
public class SimpleFactoryPattern { public static void main(String[] args) { Shoes nike = SimpleFactory.getShoes("Nike"); nike.createShoes(); Shoes adidas = SimpleFactory.getShoes("Adidas"); adidas.createShoes(); } }
abstract class Shoes{ public abstract void createShoes(); }
class NikeShoes extends Shoes{ @Override public void createShoes() { System.out.println("我是nike厂家"); System.out.println("先这样..."); System.out.println("再这样..."); } }
class AdidasShoes extends Shoes{ @Override public void createShoes() { System.out.println("我是Adidas厂家"); System.out.println("先这样..."); System.out.println("再这样..."); } }
class SimpleFactory{ public static Shoes getShoes(String arg){ Shoes shoes = null; if(arg.equals("Nike")){ shoes = new NikeShoes(); }else if(arg.equals("Adidas")){ shoes = new AdidasShoes(); } return shoes; } }
|
3.优点
比较容易理解,操作简单,外界只需要提供指定参数就可以,无需究竟如何创建及如何组织的。明确了各自的职责和权利,有利于整个软件体系结构优化。
4.缺点
违背了设计模式的ocp(对扩展开放,对修改关闭)原则.
5.使用场景